【Kotlin/Android Studio】DataBindingの方法!findViewByIdを排除する
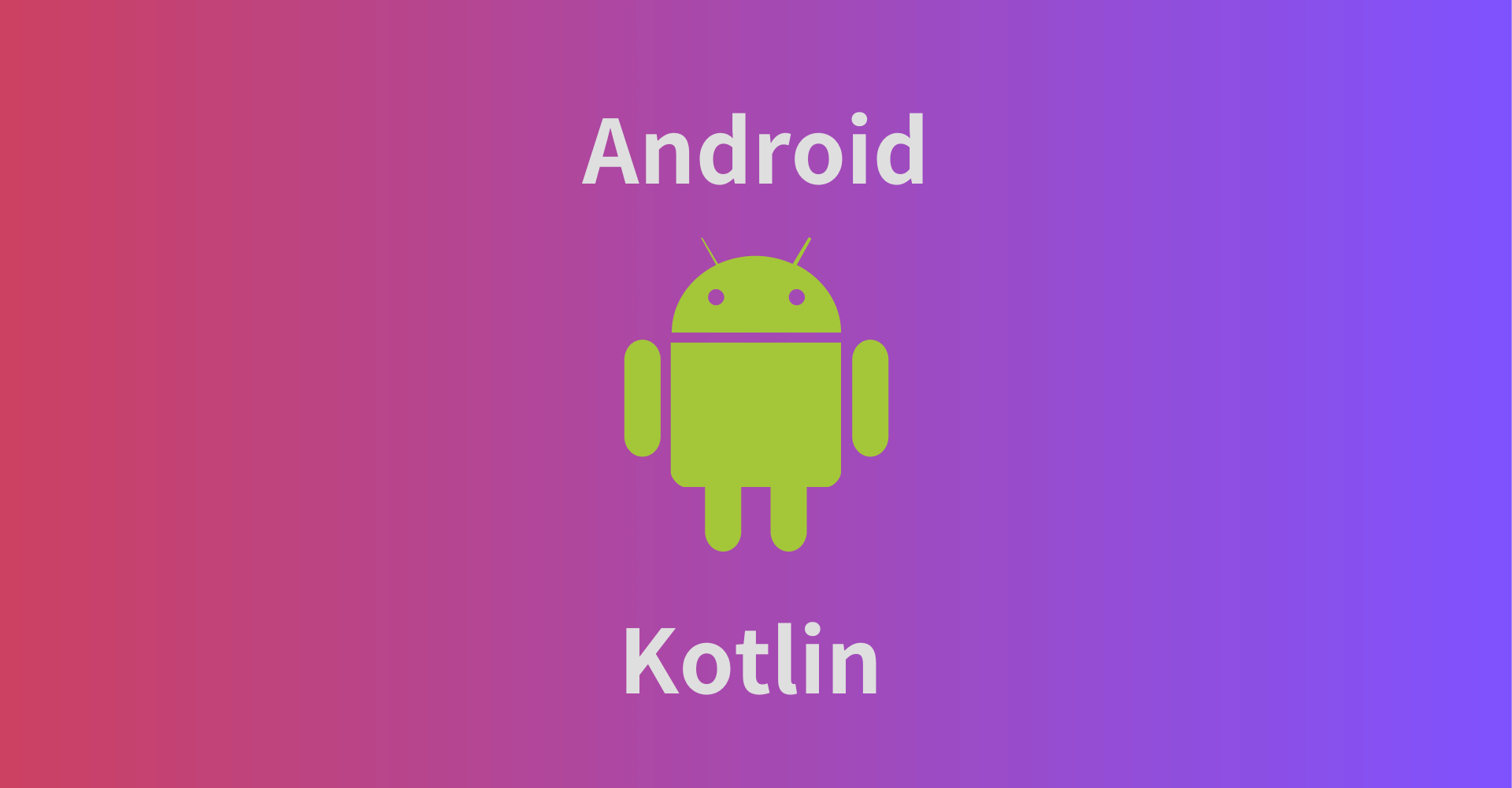
この記事からわかること
- Android StudioのDataBinding(データバインディング)を利用する方法
- findViewByIdを使用せずにViewを取得する
- データクラスをバインディングするには?
- ActivityとFragmentでの実装手順
- メモリリークを起こさないように扱う方法
index
[open]
\ アプリをリリースしました /
参考文献:公式リファレンス:3. タスク: データ バインディングを使用して findViewById() を排除する
参考文献:公式リファレンス:DataBindingライブラリ
環境
- Android Studio:Koala
- Kotlin:1.8.20
今回のプロジェクトの全体はGitHubに上げていますので参考にしてください。
DataBinding(データバインディング)とは?
DataBindingはXMLレイアウトファイルとKotlin側のコードを紐づけることができる機能でAndroid Jetpackの一部として組み込まれています。Viewの参照を取得できるViewBindingの機能に合わせて、Viewとデータモデルをバインディング(結びつける)する機能を提供しています。
そのためfindViewById
メソッドの使用が不要になり、さらにレイアウトファイルとデータモデルが紐づくことでUIの更新とデータの変更が自動的に同期し、UIコードの保守性が向上します。
DataBinding(ViewBinding)
を有効にするとXMLレイアウトファイルに紐づいたバインディングクラスが自動生成されます。生成されるバインディングクラス名はactivity_main.xml(MainActivity.kt)
ならActivityMainBinding
のようになります。
※fragment_input.xml(InputFragment)
ならFragmentInputBinding
ViewBindingとの違い
ViewBindingとの違いというより両者の違いをまとめると以下の通りです。
- ViewBinding:レイアウトとViewの参照の自動紐付け機能
- DataBinding:レイアウトとViewの参照の自動紐付け機能 + レイアウトとデータモデルの紐付け機能
DataBindingは「ViewBinding + レイアウトとデータモデルの紐付け機能」が組み込まれたフレームワークになります。レイアウトファイルとデータモデルを紐づける必要がない場合はパフォーマンスに悪影響を与える可能性があるのでViewBinding
を使用してください。
導入方法
DataBinding
を導入するには「build.gradle(Module)」内のandroid
の中にbuildFeatures { dataBinding true }
を追記して「Sync Now」をクリックします。
この際に以下のようなエラーが発生することがありますが、その場合はcompileSdk
やtargetSdk
のバージョンを確認してみてください。
Activityでの使い方
プロジェクト内で「レイアウトとViewの参照の自動紐付け機能」を利用するための手順は以下の通りです。
- レイアウトファイルを変更する
- レイアウトリソースを参照する
ViewBindingではレイアウトファイルを特に修正する必要なくバインディングクラスが自動生成されていましたが、DataBindingではレイアウトファイルを少し修正しないとバインディングクラスが自動生成されないので注意してください。
1.レイアウトファイルを変更する
DataBindingを使用したい場合はXMLレイアウトファイルのルートを<layout>タグで囲む必要があります。対象のレイアウトファイルをCode
で開いて現在のルートタグ部分で「Option + Enter」を押し「Convert to data binding layout」をクリックします。すると中身が自動的に変換されます。
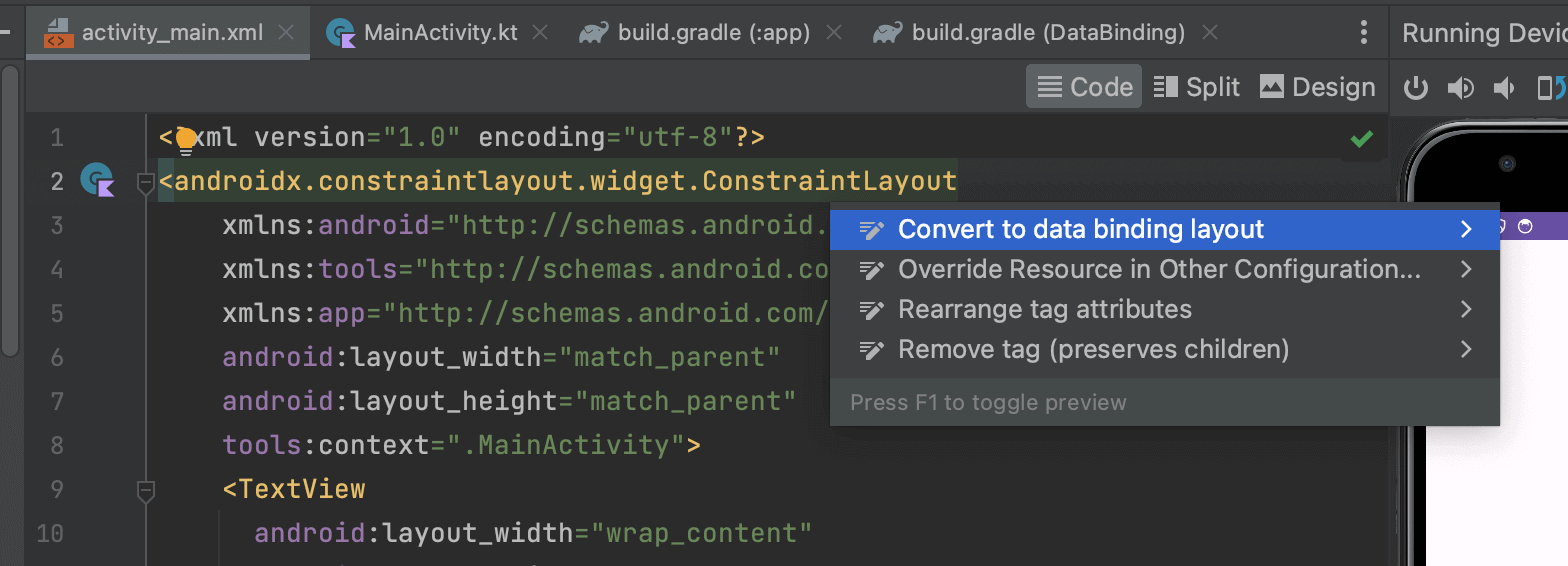
↓↓↓↓↓↓↓↓変換後↓↓↓↓↓↓↓↓
ルートに<layout>
タグが追加され、名前空間が<layout>
タグに移動しています。また<data>
タグも自動で付与されます。
2.レイアウトリソースを参照する
Activityからレイアウト内のウィジェットを参照してみます。使用するためにはまずMainActivity
クラス内にbinding
プロパティを追加します。
次にonCreate
メソッド内のsetContentView
メソッドをDataBindingUtil
を使用した以下のように置き換えます。
これで準備が整いました。binding
プロパティからウィジェットに付与したidのキャメルケースで該当のViewを取得できるようになります。例:done_button
→doneButton
データモデルの紐付けは後述しています。
Fragmentでの使い方
公式リファレンス:フラグメントでビュー バインディングを使用する
FragmentでDataBindingを使用する場合はonCreateView
メソッド内でinflate
メソッドを使用してBindingを取得し、binding.root
を返します。この際にlateinit
ではなくprivate var
で参照用のbinding
と更新用の_binding
を用意し、onDestroyView
メソッドで明示的に解放するように実装します。
lateinitではメモリリークの危険
lateinit
で実装するとメモリリークの原因になるので注意してください。これはbinding.lifecycleOwner
に指定しているライフサイクルとviewLifecycleOwner
が微妙に異なるためです。
lifecycleOwner
にviewLifecycleOwner
を指定している実装と指定していない実装を見かけますが、lifecycleOwner
にviewLifecycleOwner
を指定するのはLiveDataを使用している場合にライフサイクルを指定しないとLiveDataが動作しなくなってしまうからです。
そのためLiveData
を使用しないならlifecycleOwner
の指定は不要でメモリリークもしないのかもしれませんが、公式の実装ではFragmentではlateinit
を使用していないのでどちらにせよlateinit
を使わない方が安全だと思います。
参考記事:1. DataBinding/ViewBindingでメモリリーク
データモデルをBindingする
定義したデータモデルをViewに紐づけることでビューとデータモデル間で双方向のデータバインディングが適応され、データの変更がビューに自動的に反映されるようになります。今回は独自データクラスを作成しバインディングしていきます。
続いて<data>
タグの中に<variable>
タグを追加し、name
属性とtype
属性を以下のように記述します。タイプは各々のプロジェクト名に変換してください。
今回はデータクラスをTextViewのtextにbindingしてみます。android:text
を@={user.name}
のように@={データクラス名.プロパティ}
形式でセットします。
これでTextViewのtextがUserクラスと紐づいているので「MainActivity」内から以下のようにbinding.クラス名
で参照し、そこにデータを格納するだけでTextViewにも反映されるようになります。
動的に変化させる
EditText
から変更された値をボタンクリック時にTextViewに反映させてみたいと思います。EditText
とtextView
を追加しておきます。
コードは以下の通りになります。apply
を使って重複するbinding
を省略しています。動的に更新されたデータを反映させるにはinvalidateAll
メソッドを最後に実行します。
おすすめ記事:【Kotlin】スコープ関数の使い方!apply/also/let/run/withの違い
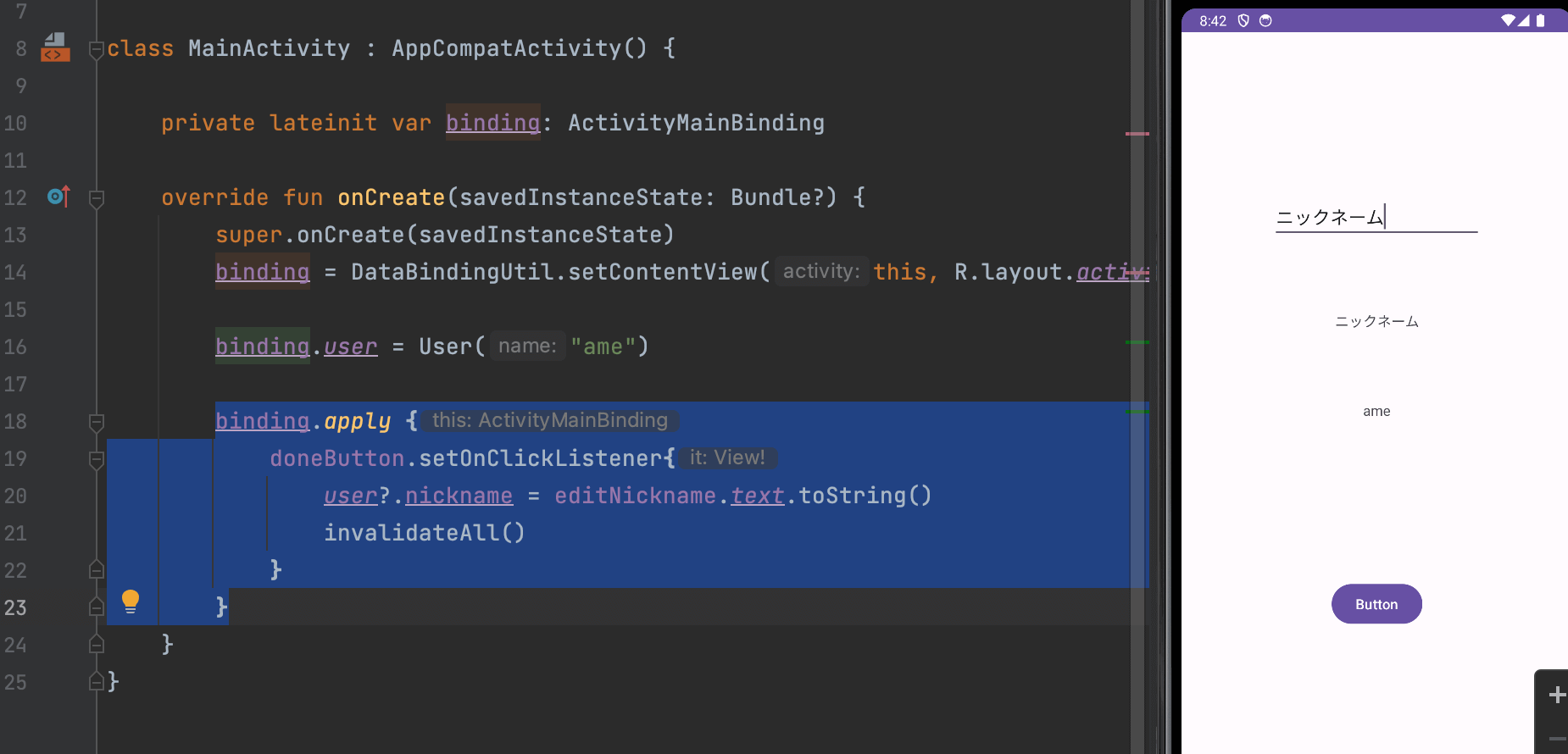
プレビュー用のデフォルト値を設ける
データに依存するように設定するとプレビューでは確認することができません。プレビューで確認できるようにしたい場合はdefault
に値を渡すことで表示させることができます。
リストやマップをバインディングする
レイアウトファイルにList
やMap
などをバインディングするにはimport
で使用するデータ型を読み込み、type
でデータ型を指定します。この際にXML構文が破綻しないように<
を使用して<
をエスケープさせる必要があります。
データを使用する際は[要素番号]
や[`キー値`]
形式で指定します。
コード側からはそれぞれの変数にデータを渡します。
リストの範囲外の対応
リスト形式で渡したデータに範囲外の要素番号を指定してしまった場合は空文字になってしまうので、代替文字を指定するには以下のように実装する ことで指定することが可能です。
使用できる演算子やキーワード
XMLレイアウトファイルでは以下の演算子やキーワードを使用することが可能です。
- 数学: + - / * %
- 文字列の連結: +
- 論理: && ||
- バイナリ: & | ^
- 単項: + - ! ~
- シフト: >> >>> <<
- 比較: == > < >= <=(< は <としてエスケープ)
- instanceof
- グループ: ()
- リテラル(文字、文字列、数値、null など)
- キャスト
- メソッド呼び出し
- フィールド アクセス
- 配列アクセス: []
- 3 項演算子: ?:
- null 合体演算子: ??
例
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。