【Kotlin/Android】ZXingでQRコードを生成とリーダーを実装する方法!
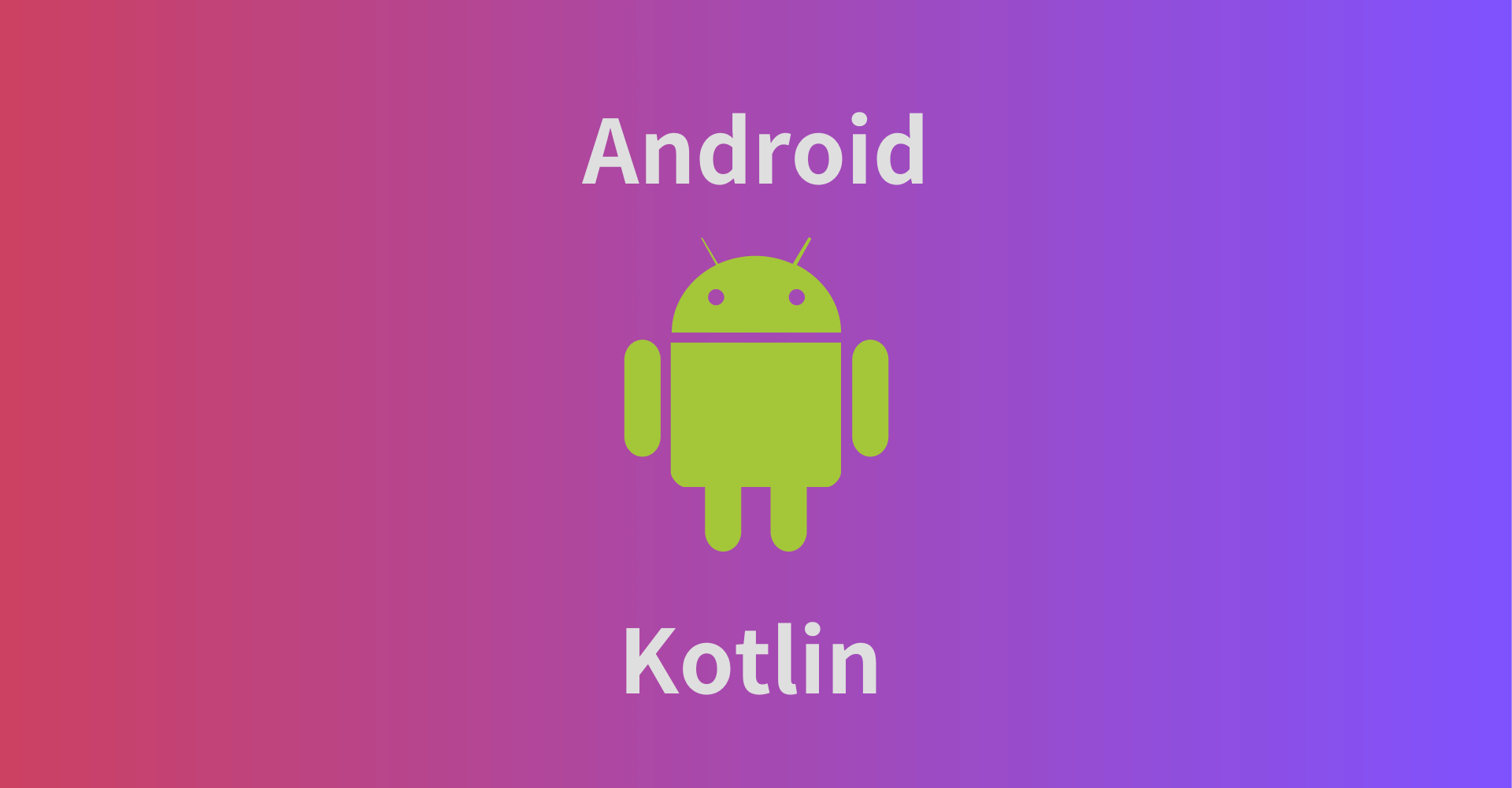
この記事からわかること
- Kotlin/ AndroidでQRコードを生成や読み取りする方法
- ZXing(Zebra Crossing)の実装と使い方
index
[open]
\ アプリをリリースしました /
環境
- Android Studio:Flamingo
- Kotlin:1.8.20
ZXing(Zebra Crossing)ライブラリ
公式リファレンス:ZXing(Zebra Crossing)
ZXing(Zebra Crossing)はAndroidアプリでバーコードやQRコードQRコードの生成や読み取りを実装できるライブラリです。
サポートしているバーコードの種類
- UPC
- EAN
- QRコード
- etc..
QRコードリーダーを実装する
ZXingを使用してQRコードを読み取るためのQRコードリーダーを実装してみます。
- ライブラリの導入
- カメラ利用のパーミッションの追加
- QRコードリーダー画面の実装
- QRコードリーダー画面の呼び出し
1.ライブラリの導入
ZXingをAndroid Studioで使用するために「bundle.gradle(Module)」に以下の文を追加して「Sync Now」をクリックします。
2.カメラ利用のパーミッションの追加
AndroidアプリからQRコードを読み取れるようにするためにはマニフェストファイルでカメラのパーミッションを定義する必要があります。「AndroidManifest.xml」に<uses-permission android:name="android.permission.CAMERA" />
を追加しておきます。
3.QRコードリーダー画面の実装
続いてQRコードリーダーを表示するための画面を定義します。XMLファイルにはDecoratedBarcodeView
を設置しておきます。
Kotlin側ではBarcodeCallback
を使用して読取結果後の処理を実装します。decodeContinuous
メソッドでコールバックをセットします。またonResume
のタイミングでresume
を実行し、QRコードリーダーを利用できるようにします。
4.QRコードリーダー画面の呼び出し
後はQRコードリーダー画面を呼び出すだけです。シミュレーターでは実際の動作確認はできないので実機などを使用して確認してみてください。

QRコードを生成する
ZXingでは簡単にQRコードを生成することが可能です。実装は以下のようになります。
生成したBitmap
をImageView
にセットすれば表示させることができます。

まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。