【Kotlin/Android】Drawableリソースの色を動的に変更する方法!
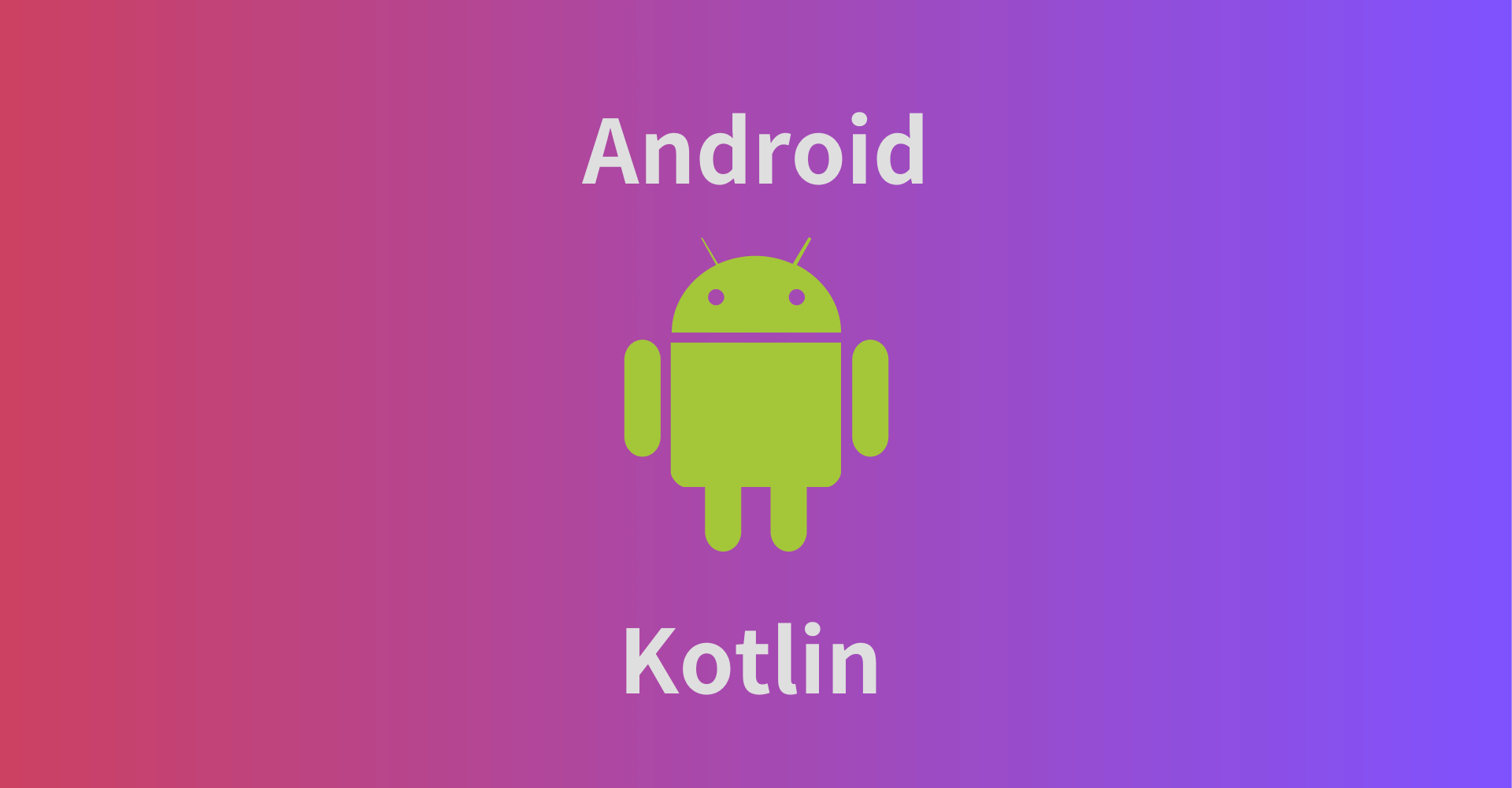
この記事からわかること
- Kotlin/Android StudioでDrawableリソースの色を動的に変更する方法
- backgroundTintメソッドの使い方
\ アプリをリリースしました /
環境
- Android Studio:Flamingo
- Kotlin:1.8.20
複数の色違いのViewやボタンを実装したい場合のDrawableリソースの定義方法と色を動的に変更する方法をまとめていきます。例えば以下のような色違いの円形Viewを実装してみます。

汎用的なDrawableリソースを定義する
まずは使い回す用の汎用的なDrawableリソースを定義します。ここでは形や必要であればサイズなどを定義していくだけです。ここでは色は適当に指定しておきます。
形の実装はshape
タグを使用することで矩形や円形だけでなく、枠線や角丸、グラデーションなど簡単に定義することが可能です。
おすすめ記事:shapeファイルの設定方法
Drawableリソースの色違いを実装する
レイアウトファイルでDrawableリソースの色違いを実装するためにはandroid:background
属性でリソースファイルを指定し、android:backgroundTint
属性でリソースファイルの色を指定することができます。
全体
コードから動的に変更する
Kotlinで動的に色を変更するにはbackgroundTintList
の値を変更します。単純にカラーコードやリソースを渡すのではなくContextCompat.getColorStateList
で指定する必要があります。
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。