【Kotlin/Android】ExpandableListViewの使い方!折り畳み(アコーディオン)ビュー
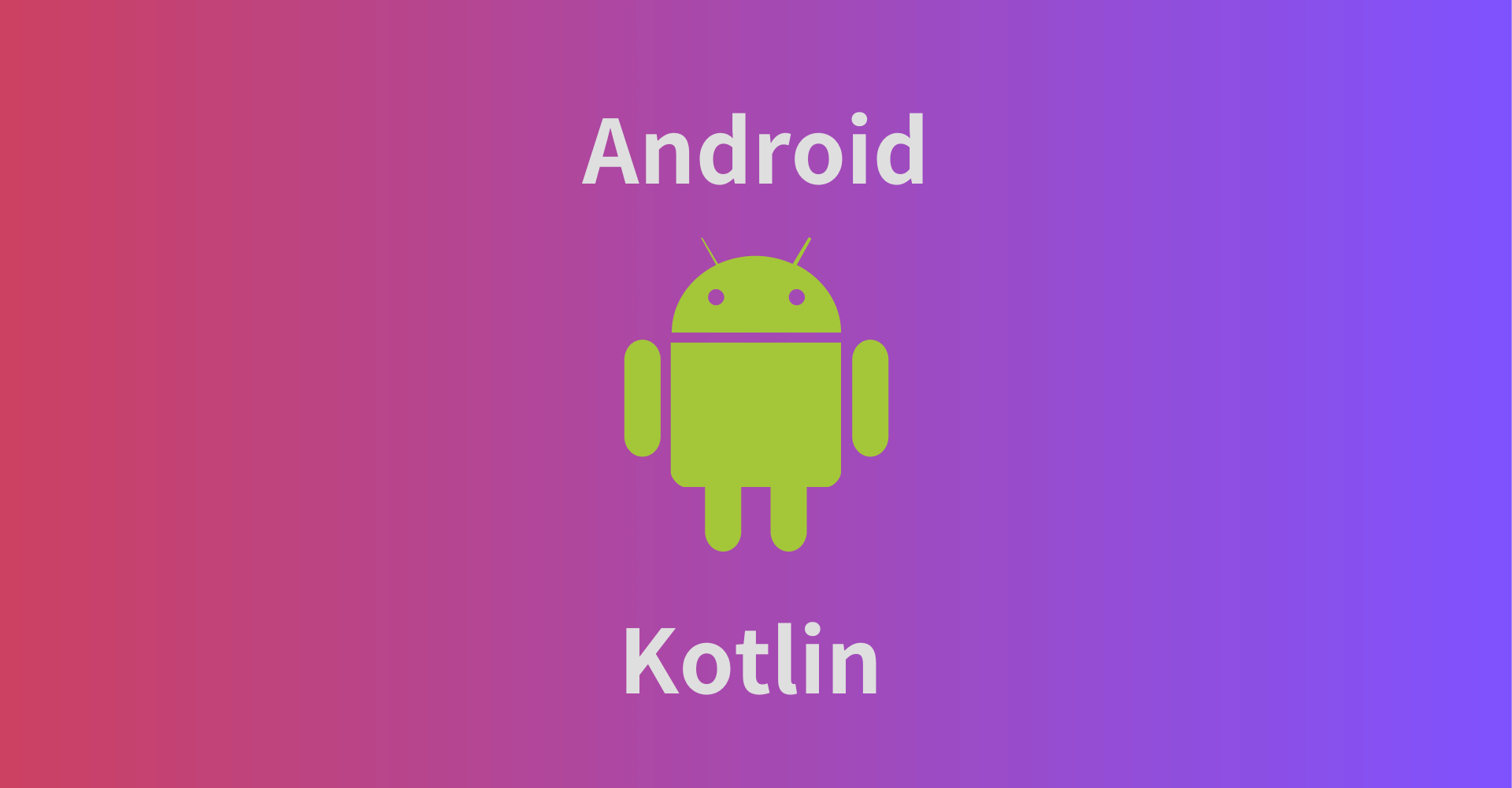
この記事からわかること
- Android Studio/KotlinのExpandableListViewの使い方
- 折り畳み(アコーディオン)を実装する方法
index
[open]
\ アプリをリリースしました /
環境
- Android Studio:Flamingo
- Kotlin:1.8.20
折り畳み(アコーディオン)ビュー
Androidアプリで以下のようにクリックすることで要素が表示/非表示されるような折り畳み(アコーディオン)ビューを実装する方法をまとめていきます。

実装方法は色々あるかと思いますがExpandableListView
を使用すると簡単に実装することができました。
ExpandableListViewとは?
ExpandableListView
は折りたたみ可能なリストを表示するために使用されるUIコンポーネントです。内部的に親要素(グループ)と子要素(アイテム)を保持し、親項目がクリックされたときに子要素を表示させることができます。
仕組みはRecyclerView
などと同じでAdapter
を介してViewとデータのやり取りを行います。
実装手順
- ExpandableListViewを設置した画面を用意
- 親要素表示用のViewをレイアウト
- 子要素表示用のViewをレイアウト
- Adapterクラスを実装
- 5.ExpandableListViewにAdapterをセット
1.ExpandableListViewを設置した画面を用意
まずはFragmentなどのレイアウトファイルにExpandableListView
を追加します。
デフォルトのUIでは左端に三角のアイコンが表示されるので不要であれば以下を指定することでアイコンを非表示にすることができます。

2.親要素表示用のViewをレイアウト
続いて親要素を表示するViewのレイアウトファイルを追加します。ここのレイアウトは自由です。
3.子要素表示用のViewをレイアウト
同じく子要素を表示するViewのレイアウトファイルを追加します。ここのレイアウトも自由です。
4.Adapterクラスを実装
続いてアコーディオンビューとデータを紐付けるためのAdapterクラスを実装します。BaseExpandableListAdapter
を継承さえて中身を実装していきます。
5.ExpandableListViewにAdapterをセット
最後にExpandableListView
にAdapter
をセットすれば完了です。
初期表示に折り畳まないようにする
デフォルトでは子要素は折り畳まれた状態ですが、初期から折り畳まないようにしたり、コードから表示させるにはexpandGroup
メソッドを使用します。
親要素(グループ)にタップイベントを追加する
親要素(グループ)にタップイベントを追加するにはsetOnGroupClickListener
を使用します。コールバックの返り値にはBoolean
型を指定します。true
にすると記述した処理だけを行い子要素を展開しません。
子要素(アイテム)にタップイベントを追加する
子要素(アイテム)にタップイベントを追加するにはsetOnGroupClickListener
を使用します。コールバックの返り値にはBoolean
型を指定します。true
にするとクリックイベントが消費されたと見なされ、他の処理は行われません。
親要素をタップした時にViewを変更する
以下動画のように展開された時に親要素のアイコンを「ー
」に変更したりなど親のViewを更新したい時があると思います。

その際は以下のように実装します。
1.アコーディオン用のデータモデルを作成
展開しているかどうかの状態を保持するデータモデルを作成します。
2.Adapterに状態変更メソッドを実装
Adapterクラスにデータモデルの展開状態を切り替えるメソッドを用意します。
3.Fragmentからタップイベントを登録
最後にFragmentからsetOnGroupClickListener
を利用して先ほどの切り替えメソッドを呼び出せば完了です。
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。