【Swift】JSONの1や0をBool(真偽値)に変換する方法!decodeIfPresent
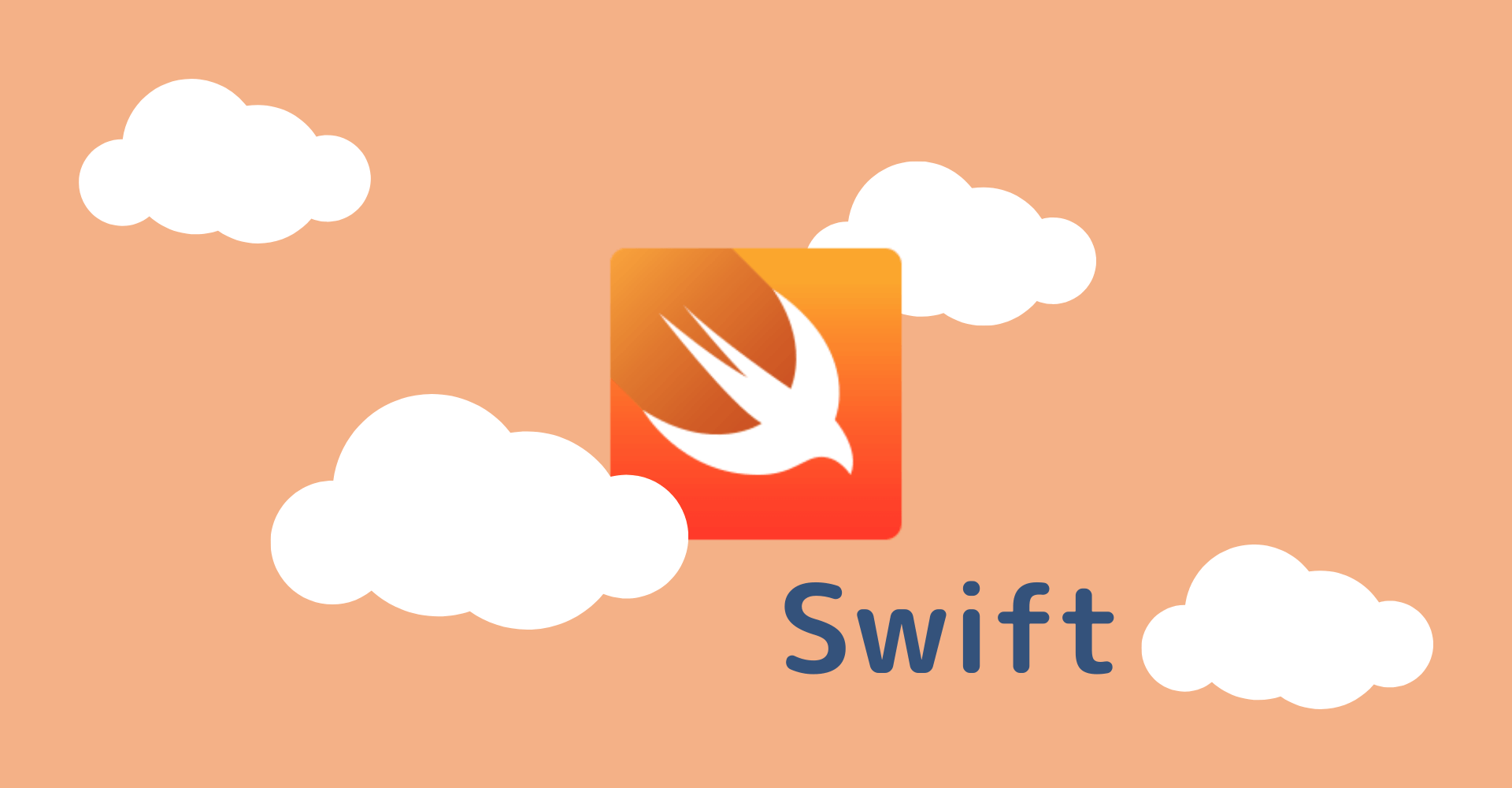
この記事からわかること
- JSONDecoderの使い方
- JSONの値が1や0の時にBool(真偽値)へ変換する方法
- APIで真偽値を扱う
- decodeIfPresentメソッドの使い方
index
[open]
\ アプリをリリースしました /
JSONデータの1や0をBool(真偽値)に変換する方法
iOSアプリからAPIと連携しJSONデータを取得しSwiftで扱えるオブジェクトに変換する際に、JSONの値が以下のように1
や0
の場合がありました。ただInt
型として扱うなら良いですがBool
型を意味する値だったので1
や0
を渡された場合にBool
型に変換する方法をまとめていきます。
JSONは以下のように値部分に数値の1
や0
が渡されます。
対象のJSON
マッピング用のクラスにBool型への変換のロジックを含ませます。変換したいキーにあったプロパティを準備しCodingKey
も定義します。CodingKey
がないと後続の処理でエラーが発生します。
JSONからオブジェクトへの変換作業は自動で行われますが、専用のイニシャライザを用意することで変換時の値を操作することが可能になります。中ではdecodeIfPresent(_:forKey:)
メソッドを使用してキー1つ1つをデコードしていきます。
マッピング用クラス
公式リファレンス:decodeIfPresent(_:forKey:)
今回は1
や0
の際にBool型へ変換していますが、値によって任意の値に変換させることも可能になります。
使用例
decodeIfPresentのtry
decodeIfPresent
メソッドは例外を投げる可能性があるメソッドです。そのためJSONの値と正しいデータ型を指定していれば成功しますが、異なるデータ型を指定すると失敗してしまいます。try
だと失敗した際にデコード自体が失敗になってしまうのでtry?
を使うことで失敗してもnilが格納されてdecodeメソッドも継続させることが可能です。
JSONに複数の値がある場合
取得できるJSONがBool型への変換だけでなく以下のようにString
型やInt
型に変換したい場合もあると思います。
この場合も実装方法はイニシャライザの中にキー1つ1つデコードする処理を記述する必要があります。構造体のプロパティに初期値があればデコードしなくてもその初期値が使用されてしまいますがデコードが不要にもできます。
エンコードする際にBool型をInt型に変換する方法
反対にSwiftのオブジェクトのプロパティがBool
型の際にJSONで1
や0
などのように任意の形に変換することも可能です。まずはオブジェクトにencode(to:)
メソッドを追加します。
ここでencode(_:forKey:)
メソッドを使用してキーに応じた変換値を指定します。今回はBoolから1/0なので上記のような実装になっています。
あとは普通にエンコード処理を実装するだけで変換することが可能です。
変換されたJSON
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。