【Swift】NSAttributedStringの使い方!文字サイズや装飾を変更する
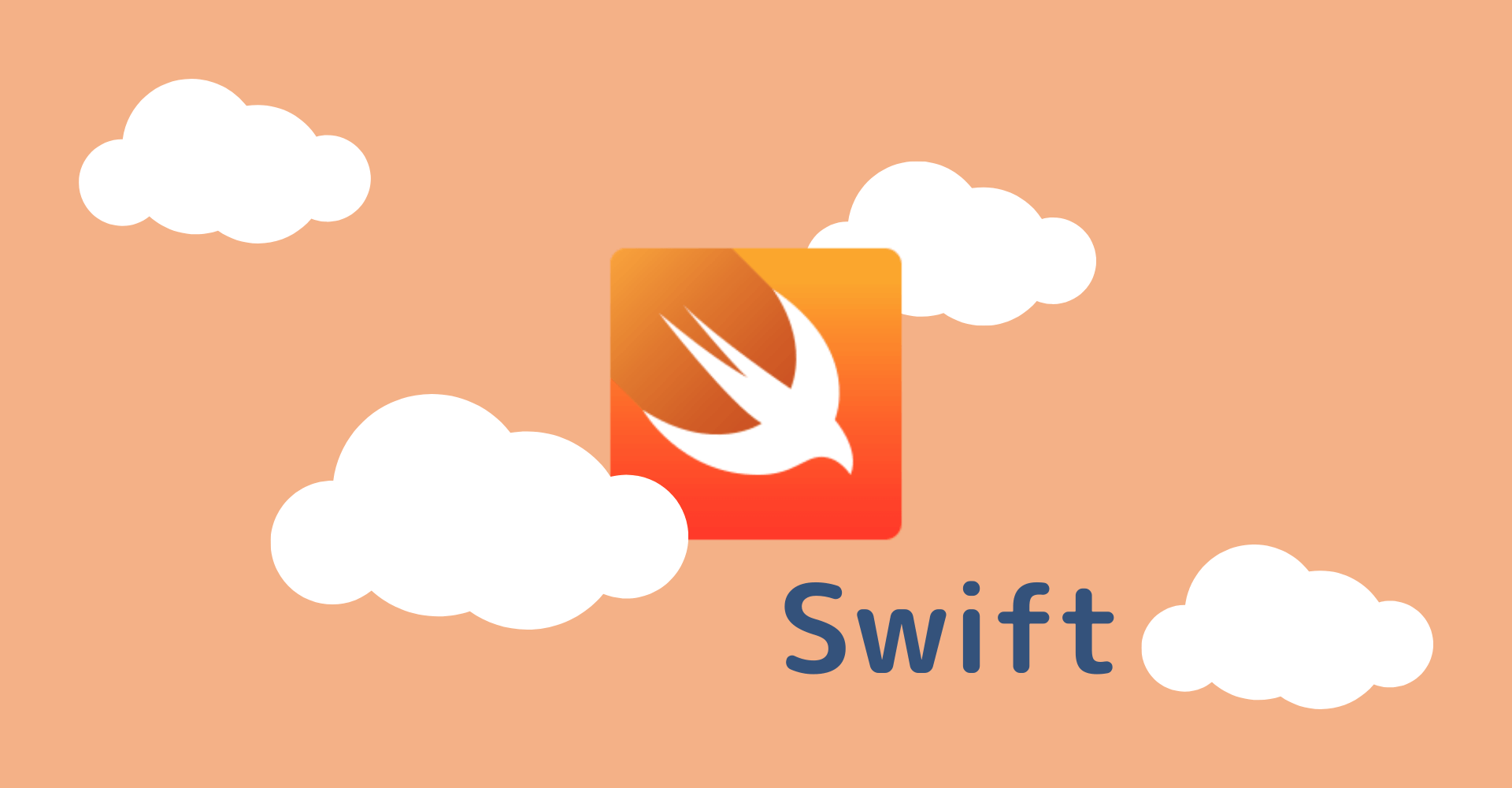
この記事からわかること
- Swift/UIKitのNSAttributedStringの使い方
- 文字サイズや行間、デザインなどを装飾する方法
- UILabelやUITextFieldのカスタマイズ方法
- NSMutableParagraphStyleやNSMutableAttributedStringとは?
index
[open]
\ アプリをリリースしました /
環境
- Xcode:15.0.1
- iOS:17.0
- Swift:5.9
- macOS:Sonoma 14.1
NSAttributedStringとは?
SwiftのNSAttributedString
はテキスト(文字)にサイズやスタイル、フォント、色、行間などの装飾を行うことができるクラスです。UILabelやUITextFieldなどのテキストのデザインをカスタマイズしたい時などに利用することができます。
使用するにはNSAttributedString
インスタンスを生成しUILabel
などのattributedText
プロパティに格納します。NSAttributedString
のイニシャライザには引数に装飾対象の文字列と装飾する属性を辞書形式で保持した[NSAttributedString.Key : Any]
型で渡します。
NSAttributedString.Keyの設定値
公式リファレンス:NSAttributedString.Key
NSAttributedString.Key
で設定できる属性は多岐にわたりますが、一部実装例を紹介しておきます。
NSMutableParagraphStyle
公式リファレンス:NSMutableParagraphStyle
NSAttributedString.Key
のparagraphStyle
にはNSMutableParagraphStyle
型で値を指定します。NSMutableParagraphStyle
はテキストの段落のスタイルを装飾するためのクラスです。調整したいプロパティに値を渡してparagraphStyle
に渡すことで反映されます。
NSMutableAttributedStringとは?
文字の装飾を行うためのクラスにはNSMutableAttributedString
もあります。NSAttributedString
と基本的な使い方は同じですが、文字全体だけでなく一部分だけ装飾を変更したりすることができるようになるのでより柔軟な装飾を行うことが可能になります。
例えばHelloだけに対して装飾を施したい場合は以下のようになります。
またNSMutableAttributedString
同士を結合することができNSAttributedString
型からも簡単にキャストすることが可能です。結合する際はappend
メソッドを使用します。
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。