【Swift UIkit】UIAlertControllerの使い方とUIAlertActionでのアラートボタン実装方法!
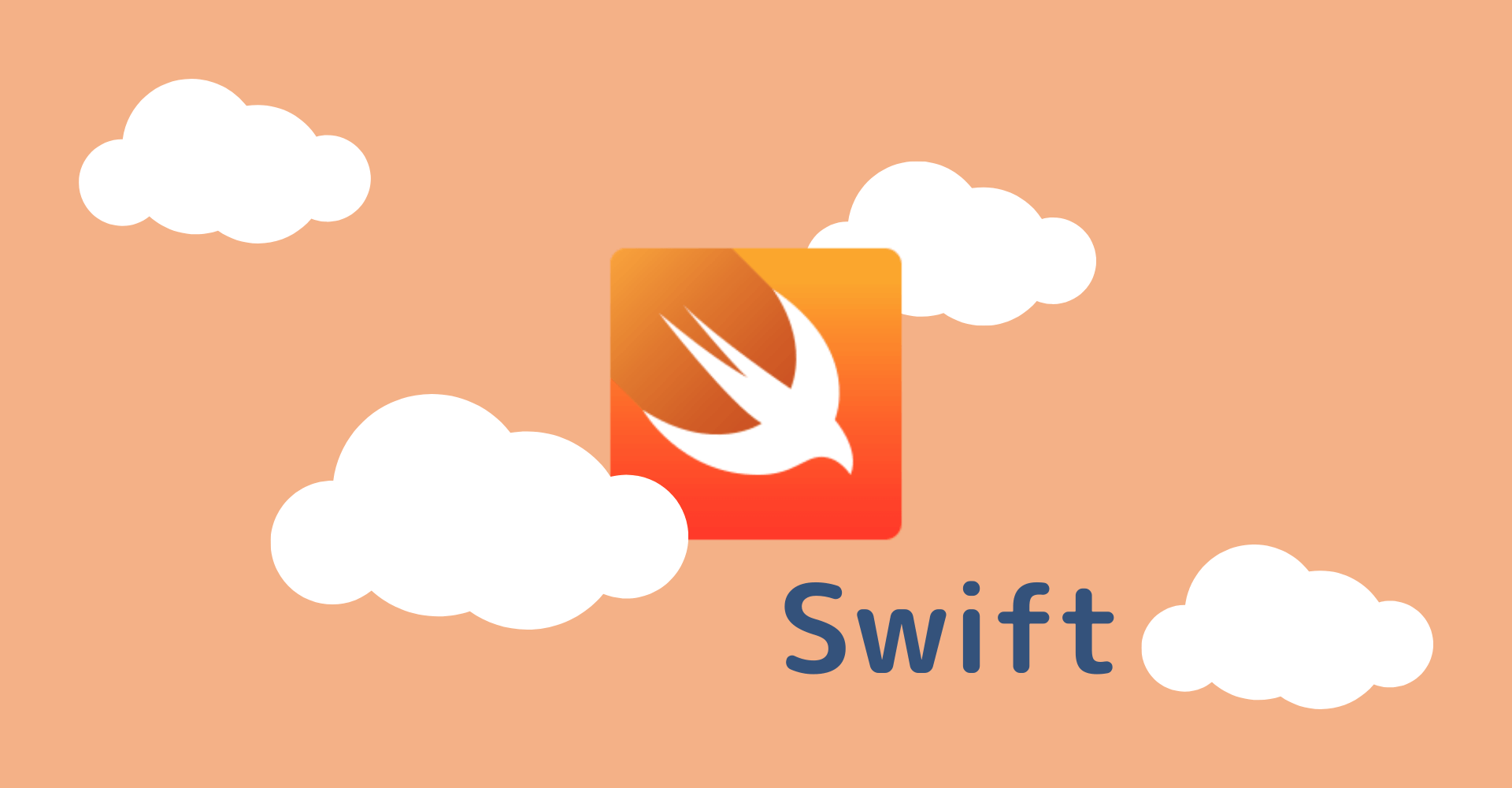
この記事からわかること
- SwiftUIKitのUIAlertControllerの使い方
- アラート表示の実装の方法
- UIAlertActionでボタンを定義する流れ
- 削除ボタンやキャンセルボタンの作り方
index
[open]
\ アプリをリリースしました /
UIKitフレームワークで以下のようなアラート機能を表示できるUIAlertControllerの使い方をまとめていきます。
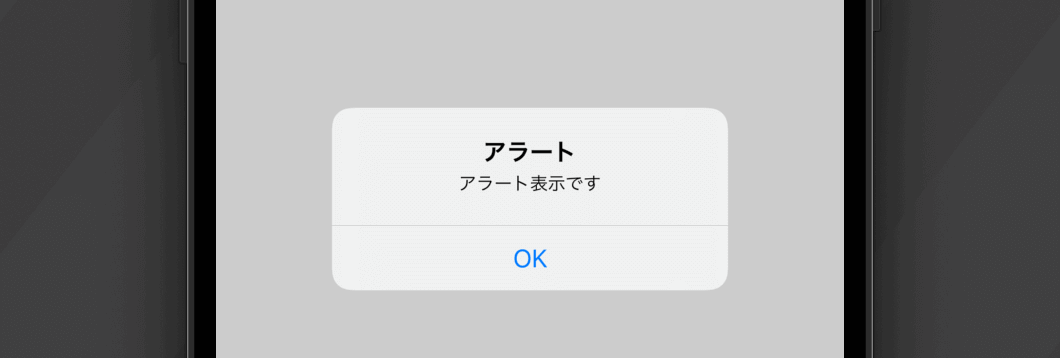
UIAlertControllerの使い方
UIAlertControllerクラスを使用してアラートを表示させる流れ
- UIAlertControllerをインスタンス化
- ボタンとなるUIAlertActionクラスをインスタンス化
- addActionメソッドでボタンを追加
- presentメソッドで表示
実装コード
UIAlertControllerをインスタンス化
最初にUIAlertController
インスタンスを生成し、アラート表示させるためのタイトルとメッセージを記述していきます。以下のようなイニシャライザが用意されているのでインスタンス化時にそのまま指定します。
ボタンとなるUIAlertActionクラスをインスタンス化
アラート表示にボタンを追加するためにはUIAlertAction
クラスを使用します。このクラスでボタンのタイトルと種類、押下された時に実行したい処理を定義します。
ボタンの種類は列挙型として定義されているUIAlertAction.Style
の中から指定します。
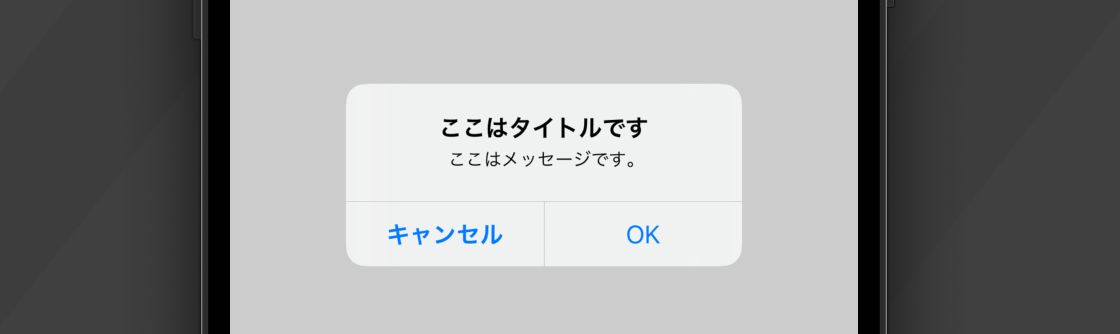
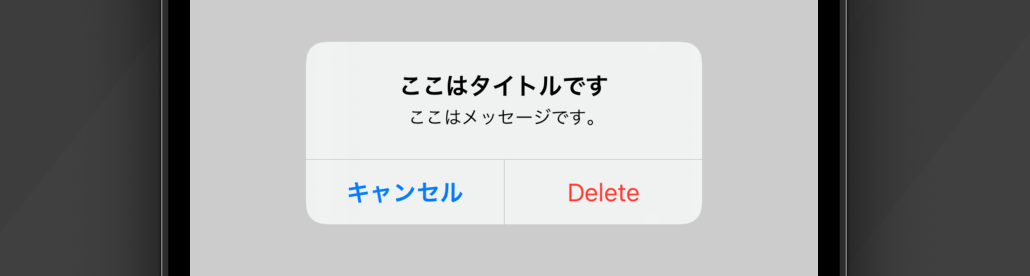
ボタンは複数実装することもできるので必要な数のUIAlertAction
インスタンスを作成しておきます。
addActionメソッドでボタンを追加
作成したボタンはUIAlertController
と紐づける必要があります。addAction
メソッドを使用することで引数に渡したUIAlertAction
インスタンスをアラートに追加することができます。
presentメソッドで表示
最後に構築したアラート表示をUIViewControllerクラスが持つpresentメソッドを使って画面に表示させます。モーダルを表示させる時などにも使用するメソッドです。
アクションシートを実装する
UIAlertControllerではpreferredStyle
にactionSheet
を使用します。以前は渡すことで画面下部から表示されるアクションシートを実装することも可能です。
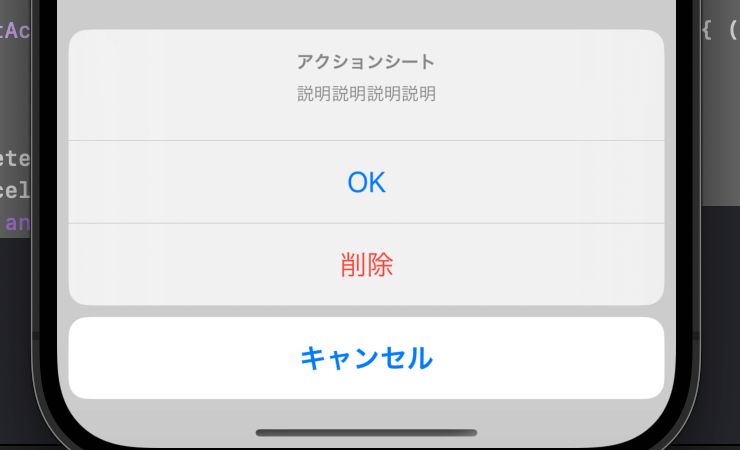
まだまだ勉強中ですので間違っている点や至らぬ点がありましたら教えていただけると助かります。
ご覧いただきありがとうございました。